ElasticSearch is really good for searching. We can use scripted field to create search fields per document. This way we don’t have to keep on updating our search filters. Instead we just click a link and the search is done for us. One such scenarios is, we have latitude and longitude and we wanted to search for other documents near that latitude and longitude. We can use the scripted field to construct a url that will make the search very easy for us.
Say our documents looked like
{
temperature: <float>,
humidity: <float>,
latitude: <float>,
longitude: <float>,
deviceName: <string>
}
Lets create a scripted field called ‘Search near me’. We will use painless script to construct a search url using the latitude and longitude value.

Select ‘Add scripted field’ under Scripted fields of the desired index. Enter a name like ‘SearchScriptedField’ and make sure the language is painless. Change the type to ‘string’, Format to ‘Url’ and Type to ‘Link’. Leave Open in a new tab as checked, this will start our search in a new tab. For Url template enter {{rawValue}} and in Label template enter ‘Search near me’. This just means each document will now show a new field called ‘Search near me’ and it will be clickable. You should land up with something like this
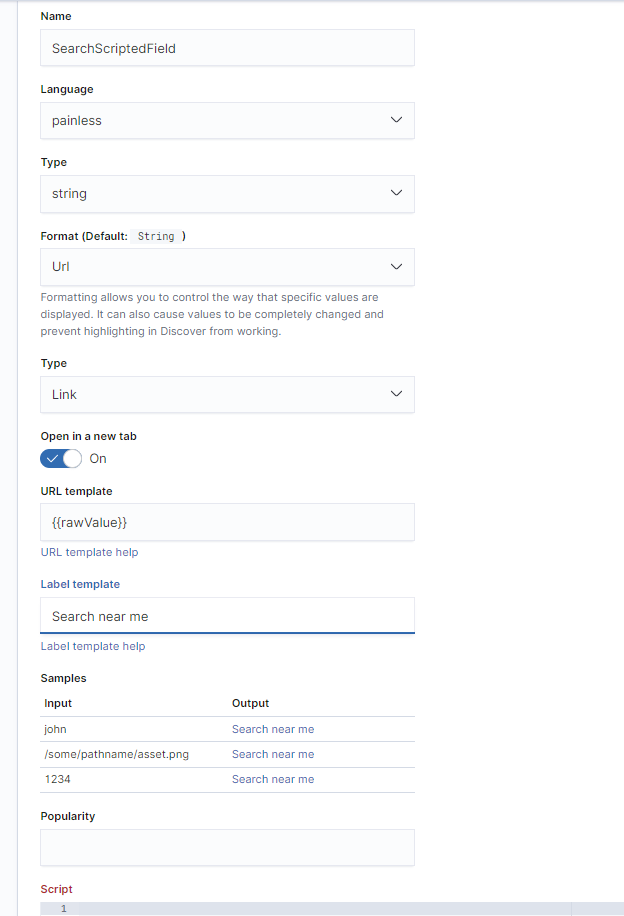
Now for the Script field. We need a script to pull out the latitude and longitude per document and construct a search url. I’ve kept the script very simple. We are going to subtract or add 0.1 to our latitude and longitude, this will become the boundary / range of our search. We also need to make sure the field exists and is not null or empty. I’ve abbreviated the return url, but you should land up with something that does a latitude and longitude range check like the below
if (doc.containsKey('latitude') && doc.containsKey('longitude') && !doc['latitude'].empty && !doc['longitude'].empty && doc['latitude'] != null && doc['longitude'] != null)
{
double lat = doc['latitude'].value;
double lon = doc['longitude'].value;
String latLow = (lat - 0.1f).toString();
String latHigh = (lat + 0.1f).toString();
String lonLow = (lon - 0.1f).toString();
String lonHigh = (lon + 0.1f).toString();
return "https:// ... range:(latitude:(gte:" + latLow + ",lt:" + latHigh + "))),..., range:(longitude:(gte:" + lonLow + ",lt:" + lonHigh + ")))) ... ";
}
return "";
I’ve found one easy way to create the return url, first perform the search in a different browser tab and add the required filters there. Then copy the url and replace the desired parameter with the strings that we have calculated and Vola!. Every time you perform a search, a new field will appear per document which will be our search link. The link text will be ‘Search near me’, and when you click the link, the browser should open a new tab with the search results.
Happy searching!